thymeleaf
- 자바 라이브러리
- 텍스트, HTML, XML, Javascript, CSS 그리고 텍스트를 생성할 수 있는 템플릿 엔젠
- JSP로 만든 기능들을 완전히 대체 가능
- spring boot에서는 jsp쓰지 말고 타임리프 쓰라고 권장
build.gradle
//타임리프뷰를 사용하려면
implementation 'org.springframework.boot:spring-boot-starter-thymeleaf'
- 위의 코드를 적으면 따로 설정 안해줘도 됨
문법
https://www.thymeleaf.org/doc/tutorials/3.0/usingthymeleaf.html
Tutorial: Using Thymeleaf
1 Introducing Thymeleaf 1.1 What is Thymeleaf? Thymeleaf is a modern server-side Java template engine for both web and standalone environments, capable of processing HTML, XML, JavaScript, CSS and even plain text. The main goal of Thymeleaf is to provide a
www.thymeleaf.org
- 공식홈페이지 문서 참고
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
- 구문 사용하려면 최상단에 xmlns:사용명(th)을 명시적으로 달기
- JSTL과 80% 유사
- 어떤 속성이든간에 타임리프 문법을 사용하고 싶으면 앞에 th를 붙여라
설명 | 표현식 | 예시 | |
변수 표현 | ${ } | ${session.user} | |
링크 | a링크에서는 @{ }로 경로 적어줌 | @{ } | @{j-won950101.tistory.com} |
fragment expression | include template 모형을 기본적으로 제공 다만 fragment 이용해서 사용 |
~{ } | frag=~{footer::#main.text()} |
텍스트 | ' ' | 'text01' | |
텍스트 결합 | + | ${str1}+${str2}, ${str1+str2} | |
문장 결합 | 참고만 하고, 특별한 경우 아니면 사용x | | | |My name is ${name}| |
조건 연산 | and, or, !, not | ${condition1} and ${condition2} | |
비교 연산 | >, <, >=, <=, gt, lt, ge, le | ${number1} > 3 |
문법 사용
- HTML 태그 내에 property명 앞에 th:를 반드시 붙임
- 붙여줘야 타임리프 식으로 해석하겠다는 뜻
설명 | 표현식 | |
th:text | 텍스트 출력 | 태그 사이의 값을 출력해줄 때 사용 |
th:value | Value 수정 | input, checkbox등 |
th:with | 변수값 지정 | 변수는 해당 태그 사이에서 언제든지 ${ }로 사용가능 |
th:if th:unless |
조건문(if, else) | else가 unless 문장으로 바뀜 |
th:each | 반복문 | 향상된 for문 (일반 for문X) |
th:href | a태그 | get 방식으로 보낼 때 href(th x) - 정적인 값, 직접 주소를 적음 th:href - a링크, 경로는 @임 |
th:block | 별도의 태그 없이 제어문 사용 | 태그를 감싸주는 태그, 의미없는 태그=가짜 태그를 만들 때 |
th:inline="javascript" | 자바스크립트에서 타임리프 사용 | js에서 사용 |
th:text
- 태그 사이의 값을 출력
- [[${변수값}]]
- Thymeleaf 3.x 에서는 태그 없이 출력 가능
<h1 th:text="${'hello world'}"></h1>
[[${'hello world'}]]
<h1 th:text="${1==1}"></h1>
[[${1==1}]]
<span th:text="${name}"></span>
th:value
- Value 수정 (input, checkbox등)
<input type="text" th:value="${val}"/>
th:with
- 변수값 지정
<div th:with="var1=${user.name}"></div>
//th:with="변수명=${값}"
실습
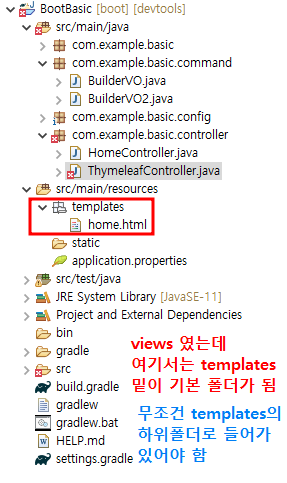
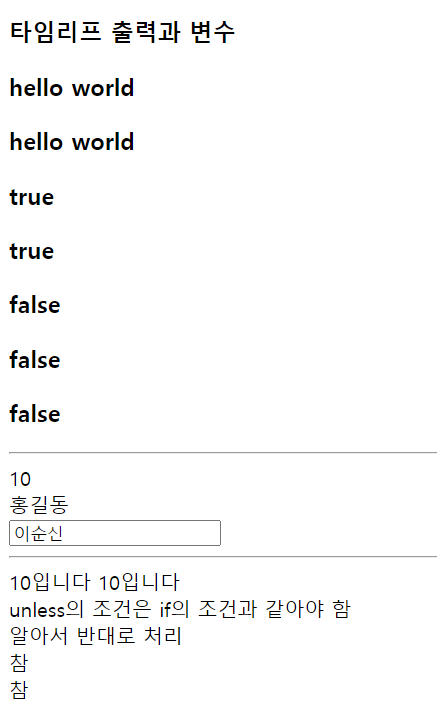
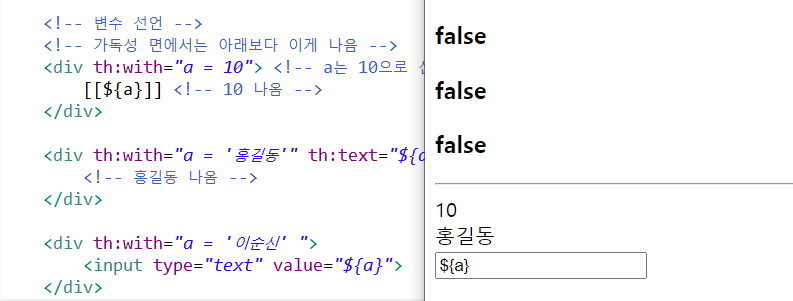
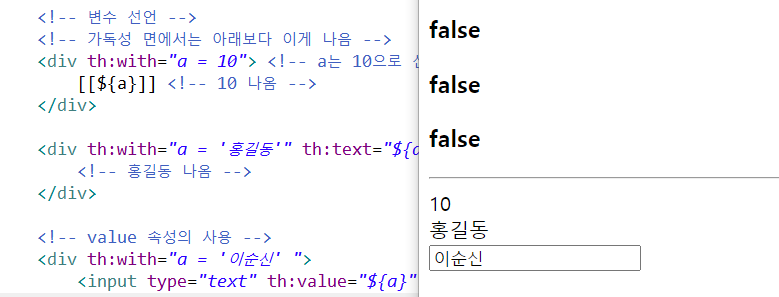
com.example.basic.controller - ThymeleafController.java
package com.example.basic.controller;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
@RequestMapping("/view")
public class ThymeleafController {
@RequestMapping("/ex01") //get방식만 허용
public String ex01() {
return "/view/ex01";
}
templates.view - ex01.html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<h3>타임리프 출력과 변수</h3>
<!-- 타임리프 문법을 사용하려면 속성 앞에 th를 붙입니다. -->
<!-- <h3 th:text="helloworld"></h3> --> <!-- 이렇게 쓰려면 공백 없어야 함 -->
<h3 th:text="${'hello world'}"></h3>
<h3>[[${'hello world'}]]</h3>
<h3 th:text="${1 == 1}"></h3> <!-- true -->
<h3>[[${1 == 1}]]</h3>
<h3 th:text="${true and false}"></h3> <!-- false -->
<h3>[[${true and false}]]</h3>
<h3>[[${'가' eq '가나다'}]]</h3> <!-- false, eq는 같냐? -->
<hr/>
<!-- 변수 선언 -->
<!-- 가독성 면에서는 아래보다 이게 나음 -->
<div th:with="a = 10"> <!-- a는 10으로 선언 -->
[[${a}]] <!-- 10 나옴 -->
</div>
<div th:with="a = '홍길동'" th:text="${a}"> <!-- a는 10으로 선언 -->
<!-- 홍길동 나옴 -->
</div>
<!-- value 속성의 사용 -->
<div th:with="a = '이순신' ">
<input type="text" th:value="${a}">
</div>
<hr/>
<!-- if문 / elseif문 자체가 없음-->
<div th:with="a = 10">
<span th:if="${a == 10}">[[${a + '입니다'}]]</span>
<span th:id="${a == 20}">[[${a + '입니다'}]]</span>
</div>
<!-- if else문: unless는 동일한 조건을 적습니다. -->
<div th:with="a = 10">
<span th:if="${a} != 10">10이 아닙니다</span>
<span th:unless="${a} != 10">unless의 조건은 if의 조건과 같아야 함<br/>
알아서 반대로 처리</span>
</div>
<!-- 3항 연산자 -->
<div th:with="a = 10">
[[${a == 10 ? "참" : "거짓"}]] <br/>
[[${a} == 10 ? '참' : '거짓']]
</div>
</body>
</html>
th:if
th:unless
- 조건문은 if만 사용해서 가능
- else가 unless문장으로 바뀜
- unless는 if와 동일한 조건문을 적음
<p th:if="${user.authType}=='web'" th:text="${user.authType}"></p>
th:each
- 반복문
<p th:each="user : ${users}" th:text="${user.name}"></p>
실습
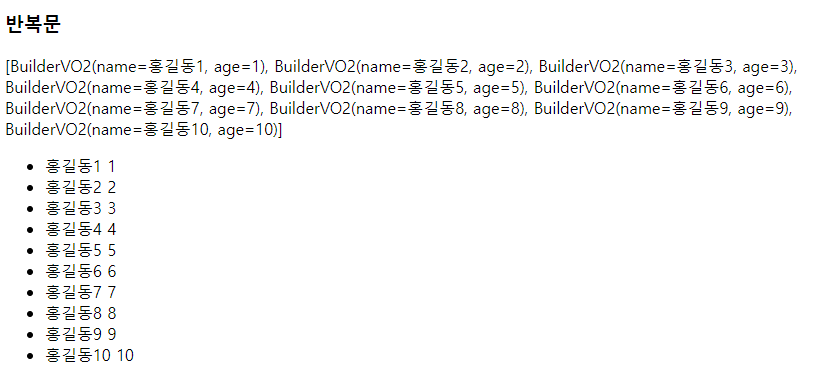
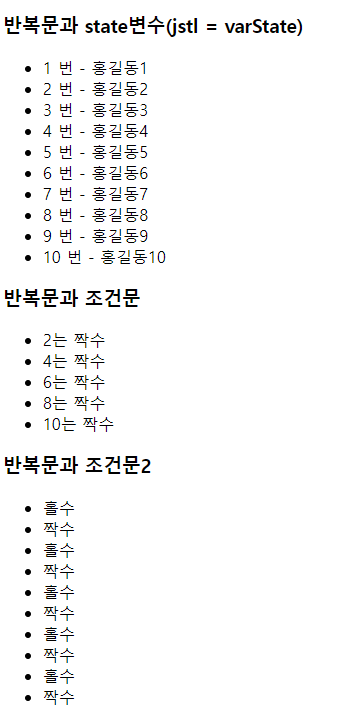
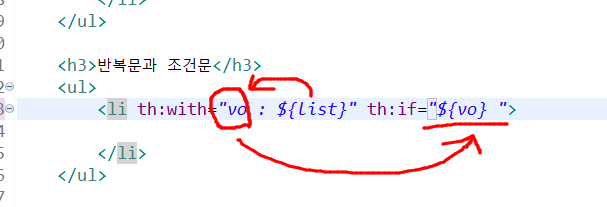
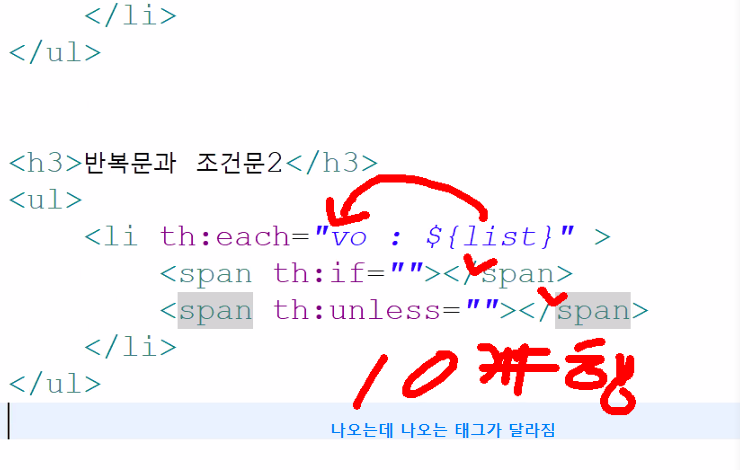
com.example.basic.controller - ThymeleafController.java
package com.example.basic.controller;
import java.util.ArrayList;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
import com.example.basic.command.BuilderVO2;
@Controller
@RequestMapping("/view")
public class ThymeleafController {
@RequestMapping("/ex02")
public String ex02(Model model) {
//사용할 가짜 데이터
ArrayList<BuilderVO2> list = new ArrayList<>();
for(int i = 1; i <= 10; i++) {
BuilderVO2 vo = BuilderVO2.builder().name("홍길동" + i)
.age(i)
.build(); //builder로 담기
list.add(vo);
}
//model
model.addAttribute("list",list);
return "/view/ex02";
}
}
- RequestMapping으로 /view/ex02 연결
- Model 객체에 담아서 화면에 뿌려줄 예정으로 BuilderVO2로 arrayList 생성
- 반복문 돌려가면서 Builder로 담기
- add()로 list에 vo를 담고, model.addAttribute로 list를 list라는 변수에 담아서 화면에서 사용
com.example.basic.command - BuilderVO2.java
package com.example.basic.command;
import lombok.AllArgsConstructor;
import lombok.Builder;
import lombok.Data;
import lombok.NoArgsConstructor;
@Data //get, set, toString
@NoArgsConstructor //기본생성자
@AllArgsConstructor //모든생성자
@Builder //빌더패턴
public class BuilderVO2 {
private String name;
private int age;
}
templates.view - ex02.html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<h3>반복문</h3>
[[${list}]]
<ul>
<!-- 이전에는 li를 감쌌는데 타임리프는 반복할 태그에 직접 반복을 걸어줌 -->
<li th:each="vo : ${list}"> <!-- list를 하나씩 뽑아서 vo라는 변수에 담음 -->
[[${vo.name}]]
[[${vo.age}]]
</li>
</ul>
<hr/>
<h3>반복문과 state변수(jstl = varState)</h3>
<!-- each에 두번째 변수를 선언하면 상태값을 담아줌 (index, count, size, current 등) -->
<ul>
<li th:each="vo, a : ${list}">
<!-- [[${a}]] -->
[[${a.count}]] 번 - [[${vo.name}]]
</li>
</ul>
// jstl과 같음
//<!-- 배열은 생성구문이 없어서 아래와 같이 적어줌 -->
//<c:set var="arr" value="<%= new int[] {1, 2, 3, 4, 5} %>" />
//
//<!-- 회전하는 방법 꼭 알아두기 -->
//<c:forEach var="a" items="${ arr }" varStatus="s">
// <!-- for(int a : arr) 이것과 같음 -->
// 인덱스번호: ${ s.index }
// 순서: ${ s.count }
// 값: ${ a }
// <br>
//</c:forEach>
<h3>반복문과 조건문</h3>
<ul>
<li th:each="vo : ${list}" th:if="${vo.age} % 2 == 0">
[[${vo.age + '는 짝수'}]]
</li>
</ul>
<h3>반복문과 조건문2</h3>
<ul>
<li th:each="vo : ${list}">
<span th:if="${vo.age} % 2 == 0">짝수</span>
<span th:unless="${vo.age} % 2 == 0">홀수</span>
</li>
</ul>
</body>
</html>
- 타임리프는 반복할 태그에 직접 반복을 걸어줌
- 3항 연산자로 list를 하나씩 뽑아서 vo에 담음
- vo(요소), a(요소의 상태를 담는 state변수) : ${list}
- js에서 foreach{(item, index) => 함수} 이거랑 같음 , vo=item이고 a=index임
th:href
- a태그
- get 방식으로 보낼 때
<a th:href=“@{경로}” >내용</a>
<!--
a태그로 값을 넘기는 방법
경로(키=값, 키=값)
경로/변수=키/변수=키(변수=값, 변수=값)
-->
<ul>
<li th:each="vo : ${list}">
<!-- <a href="test?age=${vo.age}">키값 넘기기</a> --> <!-- 가변값은 안 넘어감 -->
<!-- <a th:href="@{test?age=${vo.age}}">키값 넘기기</a> --> <!-- 이렇게 ? 써도 안 넘어감 -->
<a th:href="@{test(age=${vo.age}, name=${vo.name})}">키값 넘기기(쿼리스트링)</a> <!-- 개발자 도구에서는 쿼리스트링 형태(?이거)로 나타남 -->
<a th:href="@{ test2/{age}/{name} (age=${vo.age}, name=${vo.name}) }">키값 넘기기(쿼리파라미터)</a>
</li>
</ul>
th:block
- 가짜 태그 만들 때 태그를 감싸주는 태그
<th:block th:each="vo : ${list}"></th:block>
실습
com.example.basic.controller - ThymeleafController.java
package com.example.basic.controller;
import java.util.ArrayList;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
import com.example.basic.command.BuilderVO2;
@Controller
@RequestMapping("/view")
public class ThymeleafController {
@RequestMapping("/ex03")
public String ex03(Model model) {
//사용할 가짜 데이터
ArrayList<BuilderVO2> list = new ArrayList<>();
for(int i = 1; i <= 10; i++) {
BuilderVO2 vo = BuilderVO2.builder().name("타임리프" + i)
.age(i)
.build(); //builder로 담기
list.add(vo);
}
//model
model.addAttribute("list",list);
return "/view/ex03";
}
}
templates.view - ex03.html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<h3>타임리프 block문 = 가짜 태그</h3>
[[${list}]]
<hr/>
<!-- block은 별도의 태그를 사용하지 않고, 마치 중괄호처럼 사용하고 싶을 때 -->
<ul>
<th:block th:each="vo : ${list}">
<li>[[${vo.name}]]</li>
</th:block>
</ul>
<hr/>
<h3>타임리프 a태그</h3>
<a href="test?a=10">일반 a태그</a> <!-- 그냥 href로 정적인 값 넘기는 것은 가능 -->
<a th:href="@{test?a=10}">타임리프 a태그</a>
<!--
a태그로 값을 넘기는 방법
경로(키=값, 키=값)
경로/변수/변수(변수=값, 변수=값)
-->
<ul>
<li th:each="vo : ${list}">
<!-- <a href="test?age=${vo.age}">키값 넘기기</a> --> <!-- 가변값은 안 넘어감 -->
<!-- <a th:href="@{test?age=${vo.age}}">키값 넘기기</a> --> <!-- 이렇게 ? 써도 안 넘어감 -->
<a th:href="@{test(age=${vo.age}, name=${vo.name})}">키값 넘기기(쿼리스트링)</a> <!-- 개발자 도구에서는 쿼리스트링 형태(?)로 나타남 -->
<a th:href="@{ test2/{age}/{name} (age=${vo.age}, name=${vo.name}) }">키값 넘기기(쿼리파라미터)</a>
</li>
</ul>
</body>
</html>
- block문은 li에 직접 적지 않고, 감싸서 반복문 돌리고 싶을 때 사용 - 의미없는 태그
- a 태그에 정적인 값 넘기기 가능 - ?키=값 혹은 타임리프 문법으로 @{경로} 사용
- @{경로(키=값, 키=값)} 혹은 @{경로/{키}/{키} (키=값, 키=값)}
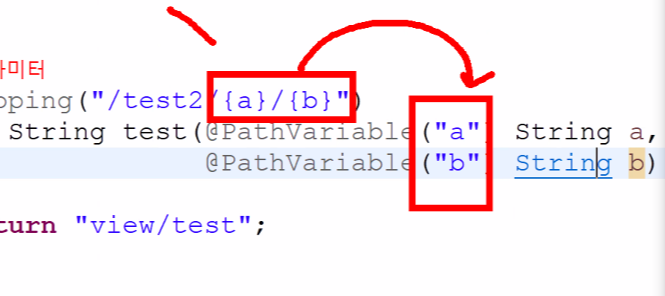
com.example.basic.controller - ThymeleafController.java
//test
//쿼리스트링 - ?으로 넘어감
@GetMapping("/test")
public String test(@RequestParam("age") int age,
@RequestParam("name") String name) {
System.out.println("test 메서드 실행");
System.out.println(age);
System.out.println(name);
return "view/test";
}
//쿼리 파라미터
@GetMapping("/test2/{a}/{b}") //3단으로 넘어오는 a,b
public String test(@PathVariable("a") String a,
@PathVariable("b") String b) {
System.out.println(a);
System.out.println(b);
return "view/test";
}
templates.view - ex03.html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<hr/>
<h3>타임리프 a태그</h3>
<a href="test?a=10">일반 a태그</a> <!-- 그냥 href로 정적인 값 넘기는 것은 가능 -->
<a th:href="@{test?a=10}">타임리프 a태그</a>
<!--
a태그로 값을 넘기는 방법
경로(키=값, 키=값)
경로/변수/변수(변수=값, 변수=값)
-->
<ul>
<li th:each="vo : ${list}">
<!-- <a href="test?age=${vo.age}">키값 넘기기</a> --> <!-- 가변값은 안 넘어감 -->
<!-- <a th:href="@{test?age=${vo.age}}">키값 넘기기</a> --> <!-- 이렇게 ? 써도 안 넘어감 -->
<a th:href="@{test(age=${vo.age}, name=${vo.name})}">키값 넘기기(쿼리스트링)</a> <!-- 개발자 도구에서는 쿼리스트링 형태(?)로 나타남 -->
<a th:href="@{ test2/{age}/{name} (age=${vo.age}, name=${vo.name}) }">키값 넘기기(쿼리파라미터)</a>
</li>
</ul>
</body>
</html>
th:inline="javascript"
- 자바 스크립트에서 타임리프 사용
<script th:inline="javascript"></script>
실습
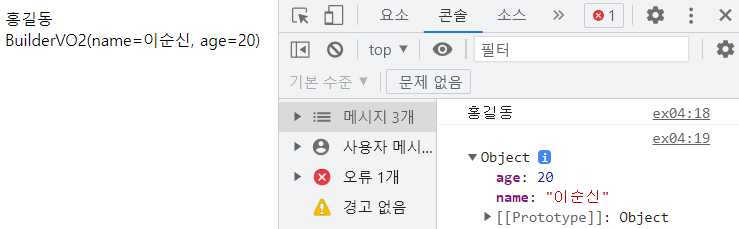
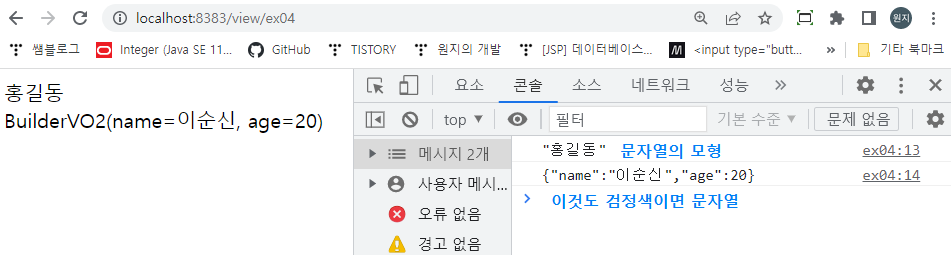

com.example.basic.controller - ThymeleafController.java
package com.example.basic.controller;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import com.example.basic.command.BuilderVO2;
@Controller
@RequestMapping("/view")
public class ThymeleafController {
//화면에서 사용
@GetMapping("/ex04")
public String ex04(Model model) {
BuilderVO2 vo = new BuilderVO2("이순신",20);
model.addAttribute(("name"), "홍길동");
model.addAttribute("vo",vo);
return "view/ex04";
}
}
templates.view - ex04.html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
[[${name}]]<br/>
[[${vo}]]<br/>
<script th:inline="javascript">
/* console.log('[[${name}]]');
console.log('[[${vo}]]'); */
var aa = '[[${name}]]';
var bb = '[[${vo}]]';
console.log(JSON.parse(aa));
console.log(JSON.parse(bb));
</script>
</body>
</html>
${#내장함수}
- Utility Object 함수들을 내장으로 가짐
- 자바의 String 내부에 기본적으로 내장되어 있는 여러 함수라고 생각하면 됨
블로그 첨부 및 구글 검색
Thymeleaf Utility Objects (1) (tistory.com)
Thymeleaf Utility Objects (1)
Author: 니용 이전 글에서 Thymeleaf의 기본적인 문법을 확인하였다면, 이번 글에서는 Thymeleaf를 더 심도 있게 활용할 수 있는 방법을 알려드리려고 합니다. Thymeleaf는 Utility Object라고 하는 함수를 기
abbo.tistory.com
실습
com.example.basic.controller - ThymeleafController.java
package com.example.basic.controller;
import java.time.LocalDate;
import java.time.LocalDateTime;
import java.util.Date;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
@RequestMapping("/view")
public class ThymeleafController {
//타임리프 내장함수
@GetMapping("/ex05")
public String ex05(Model model) {
//날짜의 형변환은 database, 자바, 화면 등 자신이 자신있는 곳에서 처리하면 됨
model.addAttribute("regdate", LocalDateTime.now() ); //날짜형, 이거 쓰세요
return "view/ex05";
}
}
templates.view - ex05.html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<h3>타임리프 내장함수</h3>
<!-- 내장함수 구글링해서 사용 -->
[[${regdate}]]
<br/>
[[${#temporals.format(regdate, 'yyyy-MM-dd')}]]
<br/>
[[${#strings.substring('홍길동', 0, 1)}]]
</body>
</html>
include 방식
- 쪼개진 파일을 참조해서 가져오는 것
- 방법은 여러가지 有
표현식 | 설명 | 예시 |
th:fragment | 조각내기 | <div th:frgament="part1"> 내용 </div> |
th:replace | 다른 파일을 include | <div th:replace="~{/include/layout01::part1}"></div> |
<div th:fragment="part1">내용</div>
<!-- part1은 외부에서 참조할 수 있는 쪼개진 파일 -->
<div th:replace="~{/include/layout01 :: part1}"></div>
1. 조각내기
- fragment로 파일을 조각내고
- replace로 include 하면 됨
2. 전체 파일 가져오기
com.example.basic.controller - ThymeleafController.java
package com.example.basic.controller;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
@RequestMapping("/view")
public class ThymeleafController {
//타임리프 include
@GetMapping("/ex06")
public String ex06() {
return "view/ex06";
}
}
templates.include - layout01.html (부분 가져오기)
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<div th:fragment="part1">
<h3>프래그먼트1</h3>
</div>
<div th:fragment="part2">
<h3>프래그먼트2</h3>
</div>
</body>
</html>
templates.include - layout02.html (파일 가져오기)
<header>
<nav>
<ul>
<li><a href="#">메뉴</a></li>
<li><a href="#">메뉴</a></li>
<li><a href="#">메뉴</a></li>
<li><a href="#">메뉴</a></li>
</ul>
</nav>
</header>
templates.view - ex06.html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<h3>타임리프 include</h3>
<!-- 1. fragment를 가져오기 ~{경로 :: 가져올 이름}-->
<div th:replace="~{/include/layout01 :: part1}"></div>
<th:block th:replace="~{/include/layout01 :: part2}"></th:block>
<!-- 2. 파일을 통째로 가져오려면 ~{경로}, 이때는 따로 fragment 안 해줬다 -->
<th:block th:replace="~{/include/layout02}"></th:block>
</body>
</html>
3. 템플릿으로 사용
com.example.basic.controller - ThymeleafController.java
package com.example.basic.controller;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
@RequestMapping("/view")
public class ThymeleafController {
//타임리프 템플릿 모형 사용하기
@GetMapping("/ex07")
public String ex07() {
return "view/ex07";
}
}
templates.include - layout02.html (템플릿 구성)
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<!-- 전체가가 하나의 템플릿화 되었음, 함수에는 매개변수 받을 수 있음 -->
<th:block th:fragment="함수(section)">
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<header>
공통 템플릿 헤더
</header>
<section th:replace="${section}">
</section>
<footer>
공통 템플릿 풋터
</footer>
</body>
</th:block>
</html>
- 템플릿으로 구성하는데 header, section, footer로 나눔
- 전체를 잡고, fragment로 전부 쪼개기
이전에는 변수명으로 받았지만 이번에는 함수로 받아서 매개변수를 넣어줄 수 있음 - 계속 바꿔줄 section에 변수명으로 그 매개변수를 넣어줌
templates.view - ex07.html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<!-- ~{파일경로 :: 템플릿함수(~{:: 선택자}) } -->
<th:block th:replace="~{/include/layout03 :: 함수( ~{:: .second} ) }">
<div id="first">
아이디 선택자 #
</div>
<div class="second">
클래스 선택자 .
</div>
</th:block>
<script>
console.log("이 파일에서만 사용")
</script>
</html>
- tiles와는 다르게 section에 템플릿이 붙는 형태
- th:replace로 조각이름 부분에 함수를 넣고, 그 안에 조각을 넣어줌(id나 class 선택자 사용)
- 이때 필요한 부분만 th:block으로 범위를 잡아서 사용함
tiles와 비교
- tiles는 템플릿에 section이 붙는 형태
2023.02.07 - [Server/Spring] - [Spring] tiles, Lombok, SpringMyweb 실습(1) - 생성 및 기본 설정
실습
templates.view - quiz01.html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<!-- layout03 템플릿에 전달되게, 아래처럼 쓰면 결합이 일어남 -->
<!-- -->
<th:block th:replace="~{include/layout03 :: 함수( ~{:: .wrap})}">
<h3>이 화면에 진입할 때 SimpleVO를 이용하여 데이터를 출력 합니다. (값은 아무거나)</h3>
<p>
회원정보확인 링크에는 quiz_result01?키=값 형태로
회원번호, 이름, 아이디 을 넘겨주세요
아래 class="wrap" 부분만 layout03 템플릿에 전달 될 수 있도록 처리하세요
</p>
<div class="wrap" th:fragment="info">
회원번호: [[${vo.num}]]<br/>
이름: [[${vo.name}]]<br/>
아이디: [[${vo.id}]]
<br/>
<!-- 타임리프 문법시 th필수 -->
<a th:href="@{quiz_result01(num=${vo.num}, name=${vo.name}, id=${vo.id})}">회원정보확인</a>
</div>
</th:block>
</html>
com.example.basic.controller - ThymeleafController.java
//quiz01- 실습
@GetMapping("/quiz01")
public String quiz01(Model model) {
SimpleVO vo = new SimpleVO("1", "제니", "jennybyj");
model.addAttribute("vo",vo);
//build로 넣어줄 수도 있음
//SimpleVO vo2 = SimpleVO.builder().num("2").name("로제").id("rose").build();
return "view/quiz01";
}
//쿼리스트링
@GetMapping("/quiz_result01")
public String quiz_result01(@RequestParam("num") String num,
@RequestParam("name") String name,
@RequestParam("id") String id,
Model model) {
model.addAttribute("num", num);
model.addAttribute("name", name);
model.addAttribute("id", id);
return "view/quiz_result01";
}
templates.view - quiz_result01.html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<h3>회원 정보 확인</h3>
회원번호: [[${num}]]<br/>
이름: [[${name}]]<br/>
아이디: [[${id}]]<br/>
</body>
</html>
오늘 하루
기억에 남는 부분
-
-
어려운 부분
-
-
문제 해결 부분
-
-
'Server > Spring boot' 카테고리의 다른 글
[Spring Boot] BootMyweb (3) - 파일 업로드, 불러오기, selectKey (0) | 2023.02.21 |
---|---|
[Spring Boot] RestAPI, 부메랑, @RestController, @RequestBody, CrossOrigin (0) | 2023.02.16 |
[Spring Boot] BootMyweb (1) - 관리자 홈페이지 / 기본 연결, 검색&Paging (0) | 2023.02.15 |
[Spring Boot] Valiadation(유효성 검사), MyBatis (0) | 2023.02.14 |
[Spring Boot] 설치 및 개발환경 구축, 기본, gradle, 개별적인 bean설정, TestCode, Builder(Lombok) (0) | 2023.02.10 |